Trees, a fundamental data structure in computer science, find their applications in numerous domains, from network topologies to organizational hierarchies. While there are various methods to represent and manipulate trees, the Prufer Code stands out due to its succinctness and elegance. This sequence of integers uniquely captures the essence of a labeled tree, making it a powerful tool for many computational tasks. In the vast arena of programming, C language offers efficiency and control, making it an apt choice for delving deep into the intricacies of the Prufer Code. This article embarks on a journey through the C implementation of decoding a Prufer Code, unveiling the algorithm’s beauty and precision. Join us as we dissect the code, offering insights and deepening the understanding of this mathematical marvel.
Arthur Cayley’s Groundbreaking Proof and Heinz Prüfer’s Novel Contribution
The realm of mathematics was illuminated when Arthur Cayley unveiled that for any given ‘n’ vertices, the number of possible labeled trees is nn−2. Later, Heinz Prüfer also proved the same theorem but through an ingenious route, leading to the discovery of what’s now known as Prüfer codes. These are (n−2)-tuples derived from a sequence of integers ranging from 1 to n-2. Heinz Prüfer’s proof wasn’t just an academic exercise; it provided a systematic method to construct any labeled tree using its corresponding Prüfer code.
Understanding the Algorithm
To grasp how this transformation from Prüfer codes to trees takes place, here’s a comprehensive breakdown of the algorithm:
- Initialization Phase:
- Begin by setting up an array that will record the degree (number of edges) for each node;
- Ensure the nodes are numbered sequentially from 1 through n+2.
- Degree Augmentation:
- Whenever a node number appears in the Prüfer code, raise its degree by one;
- Subsequently, increase the degree of every node in the sequence by one.
- Edge Creation Process:
- Iteratively go through each node number present in the Prüfer code. For every such occurrence:
- Identify the node with the smallest number that still holds a degree of 1;
- Create a bond, or edge, between this node and the one indicated in the Prüfer code;
- As a result of this bond, reduce the degree count for both nodes by one.
- Iteratively go through each node number present in the Prüfer code. For every such occurrence:
- Final Touch:
- At the conclusion of the process, two nodes will remain, each with a singular degree. Connect these two nodes with an edge, giving the tree its final structure.
Key Takeaways and Recommendations
- Prüfer codes are a brilliant representation that allows for efficient storage and reconstruction of trees;
- When reconstructing a tree using this algorithm, always pay attention to node degrees. Managing these correctly is crucial for the successful execution of the process;
- Understanding the mechanics of this algorithm can aid in various computer science applications, especially in areas like network design and data compression.
A Deep Dive into the Prufer Code Implementation in C
A Prufer Code is a sequence of integers that represents a unique labeled tree. Using this code, it’s possible to derive a tree and vice versa. Let’s explore an insightful representation of how to decode a Prufer Code into a tree using the C language.
1. The Core Structure and Function
The structure that models an edge between two nodes in the tree is defined as:
typedef struct {
unsigned int start;
unsigned int end;
} Edge;
Here, start and end denote the two nodes connected by an edge.
The primary function to convert a Prufer Code to a tree is decode_prufer(). This function accepts the Prufer Code as an array of unsigned integers along with its length. It outputs the tree as an array of edges.
2. Memory Allocation and Initialization
Inside the function, memory is dynamically allocated for the tree and for an array of node degrees. The node degrees store the number of edges connected to each node. If memory allocation fails, the function cleans up and exits early:
Edge *tree = (Edge *)malloc((num_nodes - 1) * sizeof(Edge));
unsigned int *node_degrees = (unsigned int *)calloc(num_nodes + 1, sizeof(unsigned int));
if (!tree || !node_degrees) {
free(tree);
free(node_degrees);
return NULL;
}
3. Determining Node Degrees
The function first determines the degree of each node based on its occurrences in the Prufer Code. Since a Prufer Code of length ‘L’ represents a tree with ‘L + 2’ nodes, every node starts with a degree of 1, which is then incremented based on its occurrences:
for (i = 0; i < code_length; i++) {
node_degrees[code[i]]++;
}
for (i = 1; i <= num_nodes; i++) {
node_degrees[i]++;
}
4. Building the Tree
The function proceeds to construct the tree by linking nodes with degree 1 to nodes in the Prufer Code. After each edge is added, the degrees of the involved nodes are decreased:
for (i = 0; i < code_length; i++) {
for (j = 1; node_degrees[j] != 1; j++);
tree[edge_index].start = j;
tree[edge_index].end = code[i];
node_degrees[j]--;
node_degrees[code[i]]--;
edge_index++;
}
5. Finalizing the Tree
The last step is to connect the remaining nodes with a degree of 1:
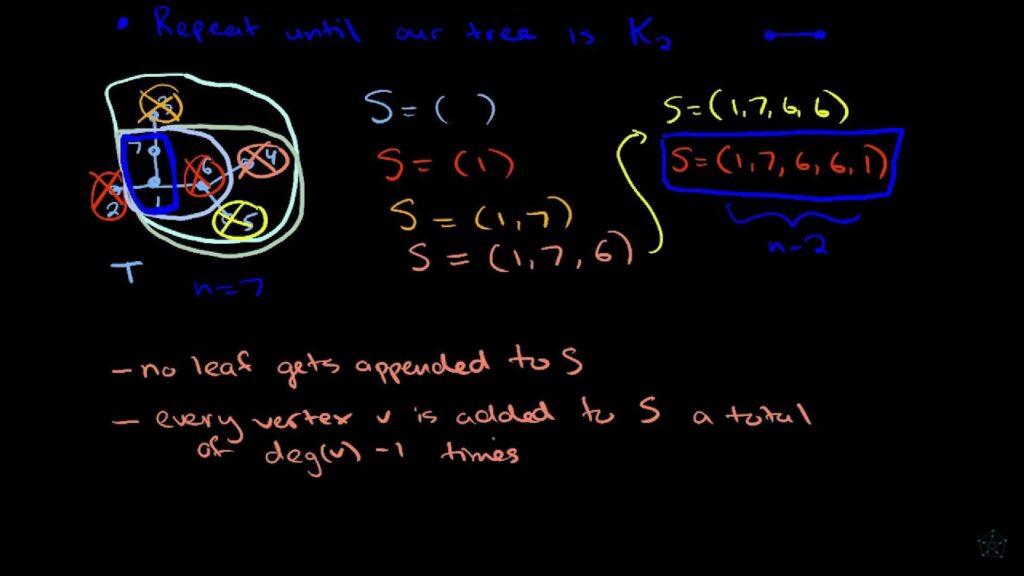
for (i = 1; node_degrees[i] != 1; i++);
for (j = i + 1; node_degrees[j] != 1; j++);
tree[edge_index].start = i;
tree[edge_index].end = j;
6. Sample Program
To visualize this, consider a sample program that utilizes the decode_prufer() function to construct a tree:
#include <stdio.h>
#include <stdlib.h>
int main(void) {
unsigned int prufer_code[] = {1, 1, 1, 1, 6, 5};
size_t code_length = sizeof(prufer_code) / sizeof(unsigned int);
Edge *resulting_tree = decode_prufer(prufer_code, code_length);
for (unsigned int i = 0; i < code_length + 1; i++) {
printf("(%u, %u)\n", resulting_tree[i].start, resulting_tree[i].end);
}
free(resulting_tree);
return 0;
}
When executed, this program yields the following tree structure:
(2, 1)
(3, 1)
(4, 1)
(7, 1)
(1, 6)
(6, 5)
(5, 8)
In conclusion, the Prufer is a powerful tool to represent trees compactly, and its decoding implementation in C is a fascinating demonstration of its efficiency. With a clear understanding of this algorithm, one can easily manipulate trees and harness its potential in various applications.
Conclusion
Understanding and implementing the Prufer Code in C showcases the beauty of computational mathematics and its intersection with software development. This unique representation provides an elegant and compact means to model, enabling efficient storage, communication, and manipulation of complex structures. The detailed decoding process exemplifies the importance of algorithms in computer science and their real-world implications. Mastery over such techniques not only enhances programming skills but also provides a solid foundation for addressing complex computational challenges. As our technological landscape becomes more intricate, tools like it remind us of the value of foundational algorithms and their timeless relevance in evolving digital ecosystems.