Selenium is a powerful tool used primarily for web application testing. It allows testers to write scripts in several programming languages such as Java, Python, and C#. One of the critical capabilities of Selenium is the execution of JavaScript code in the context of the currently selected frame or window. This is especially useful for interacting with web elements in ways that normal Selenium methods might not allow.
Why Execute JavaScript through Selenium?
Before delving into the ‘how’, it’s vital to understand the ‘why’. There are several reasons:
- Direct Element Interaction: Sometimes, web elements may not be directly accessible or interactable using standard Selenium methods. JavaScript execution provides an alternative path;
- Page Manipulation: JS can dynamically change webpage content, making it useful for testing dynamic behaviors or setting up specific test conditions;
- Data Extraction: Extracting information that might not be readily available through typical Selenium methods becomes possible.
Benefits and Precautions
- Flexibility: Directly executing JS provides testers with unparalleled flexibility in testing scenarios that would be otherwise challenging with standard Selenium methods;
- Speed: Sometimes, using JS can be faster than traditional Selenium methods, especially when dealing with complex DOM manipulations or interactions;
- Caution: Relying too heavily on JavaScript executions can make your tests brittle, as they may bypass typical user interactions. Always ensure your tests reflect real-world scenarios as closely as possible.
Let’s delve into how you can execute JavaScript within Selenium in various languages:
Java
In the Java programming realm, Selenium offers the WebDriver tool, enabling the execution of JavaScript through the`JavaScriptExecutor` interface. By casting your WebDriver instance to a `JavaScriptExecutor`, you can utilize the `executeScript()` method. This method executes the JavaScript you pass to it and returns an `Object`.
Here’s an example of how you can fetch the title of a web page using JS and Selenium in Java:
String title = ((JavascriptExecutor) driver).executeScript("return document.title;").toString();
Python
Python’s Selenium bindings simplify the process even more. The WebDriver in Python already comes with the `execute_script()` method, making it straightforward to run JavaScript commands.
Here’s how you can get the title of a web page using JS and Selenium in Python:
title = driver.execute_script("return document.title;")
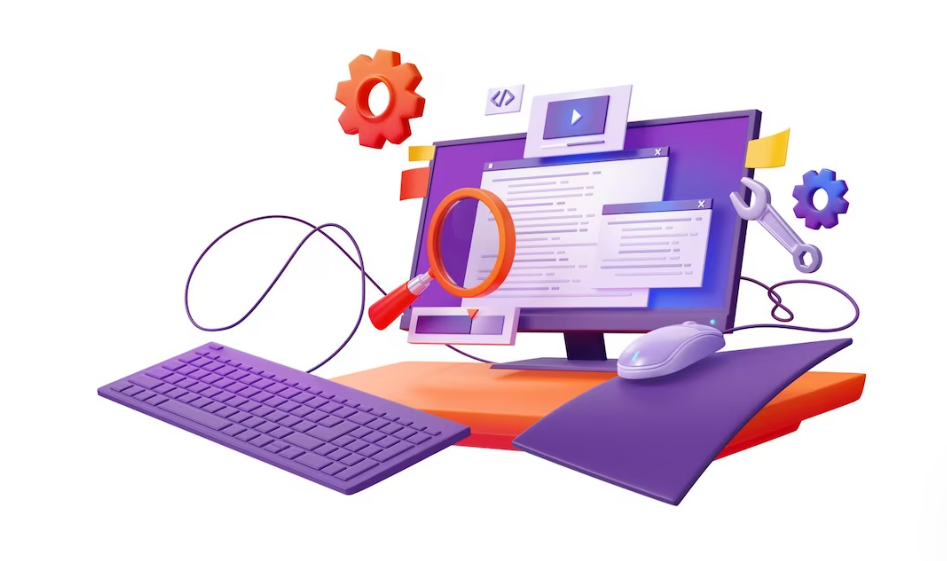
C#
For those using C#, the WebDriver can be cast to an `IJavaScriptExecutor`. This interface provides the `ExecuteScript()` method, which, like in Java, allows you to execute JavaScript and returns an `Object`.
Here’s an example in C#:
String title = ((IJavaScriptExecutor) driver).ExecuteScript("return document.title;").ToString();
Conclusion
Executing JavaScript in your Selenium scripts can open a myriad of opportunities, from manipulating web elements to extracting information that might not be readily accessible using regular Selenium methods. Whichever programming language you use, Selenium offers a straightforward method to run your JavaScript seamlessly. For those keen on exploring more in-depth topics in programming, there’s another article discussing the implementation of a Spanning Forest in C.