When diving into the world of Python programming, you’re bound to come across a multitude of tasks that require precision and nuance. One such task is zero padding a string. Whether you’re formatting numbers, aligning text, or preparing data for analysis, understanding the intricacies of zero padding is essential. Let’s embark on this enlightening journey together!
What is Zero Padding?
At its core, zero padding refers to the practice of adding zeros at the beginning of a string to make it reach a specific length. Think of it as giving a string some extra cushioning, ensuring it’s just the right size for your needs.
Why Use Zero Padding?
- Uniformity: Ensures data maintains a consistent format, especially useful for tabulated data;
- Aesthetic Reasons: Helps in making output visually consistent and aligned;
- Data Preparation: Vital for certain algorithms that require input strings of specific lengths.
Python’s Inbuilt Zero Padding Methods
Python, being the versatile language it is, provides inbuilt methods to help with zero padding.
Using the zfill() method
Python strings come equipped with a method called zfill(). It’s like they were born ready to pad!

Using f-strings in Python 3.6+
The advent of f-strings brought about a concise way to format strings, zero padding included.

Custom Padding Techniques
While Python’s inbuilt methods are powerful, sometimes you crave that bespoke touch. Let’s craft our own zero padding functions!
Using String Concatenation
Old school, but gold school. It’s like using a vintage typewriter in a world of laptops.

Using Python’s ljust/rjust Methods
These methods adjust the string’s alignment by adding padding characters. We’re just flipping the script by using zeros!

Zero Padding Applications in Real-world Scenarios
Understanding the “how” is splendid, but grasping the “why” is transcendental.
Database Management
Imagine a product database where product IDs are of fixed length. Zero padding ensures every ID aligns perfectly.
File Naming Conventions
In automated file generation systems, especially ones that name files sequentially, zero padding keeps filenames neat and orderly.
Common Pitfalls and Precautions
The road to mastering zero padding is not without its bumps. Here’s how to avoid them:
- Over Padding: Ensure the width specified for padding doesn’t exceed the intended size;
- Stripping Relevant Zeros: Be cautious when removing leading zeros, you might strip meaningful data.
Bellman Ford Algorithm in C
While zero padding is a cornerstone in string manipulation, let’s momentarily shift our attention to another intriguing domain: the Bellman Ford algorithm implemented in C. A key algorithm for finding the shortest path in weighted graphs, it’s renowned for its capacity to handle negative weight cycles.
Key Features of Bellman Ford in C:
- Versatility: Unlike Dijkstra’s, Bellman Ford can process graphs with negative weight edges;
- Iterative Approach: The algorithm relaxes all the edges, for every vertex, ‘V-1’ times (where V is the number of vertices).
Here’s a snippet of how one might begin implementing Bellman Ford in C:
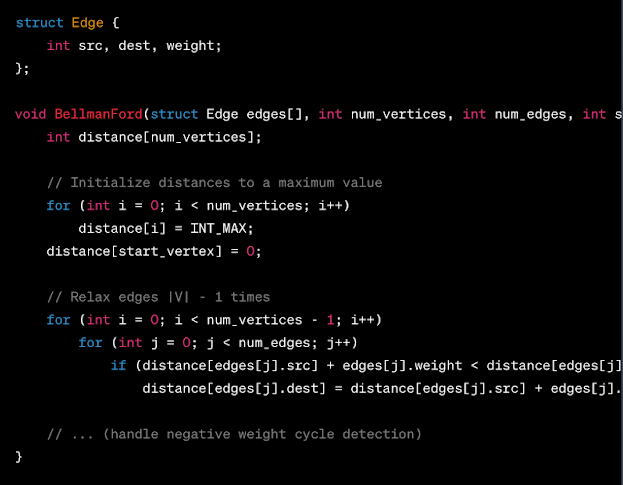
Remember, while this gives you a starting point, a full implementation requires handling negative weight cycles and possibly providing a detailed output of the shortest path.
Relevance to Zero Padding? While the Bellman Ford algorithm and zero padding seem worlds apart, they’re both essential tools in a programmer’s kit, exemplifying the vast spectrum of tasks one might tackle in the coding realm.
Conclusion
Zero padding, though seemingly simple, carries profound importance in Python programming. From data manipulation to aesthetic alignment, its applications are vast. Mastering it not only enhances your coding prowess but also prepares you for a myriad of real-world scenarios.
FAQs
Primarily, it ensures strings maintain a consistent format.
No, Python offers other methods like f-strings and ljust/rjust, as well as custom techniques.
Indirectly, yes. You’d typically convert the data to a string first.
No, it only alters the string’s appearance.
You can use Python’s lstrip() method to remove leading zeros.