In the ever-evolving realm of C++ programming, the Boost library holds a notable position. One of its prominent features, BOOST::ENABLE_SHARED_FROM_THIS, has revolutionized the way developers manage shared pointers. This article delves into its functionalities, benefits, and practical applications, ensuring you grasp the essence of this powerful tool.
Understanding Shared Pointers
Before diving into the specifics, it’s crucial to get a hang of shared pointers. Think of shared pointers as guardians; they ensure that an object remains alive as long as there’s a reference to it.
Why Use Shared Pointers?
- Memory Management: Helps in automating memory management, reducing the chances of memory leaks;
- Multiple References: Can have multiple pointers referencing the same object;
- Automatic Deletion: The object is automatically deleted when the last shared pointer referencing it is destroyed.
Diving into BOOST::ENABLE_SHARED_FROM_THIS
This Boost feature is a lifesaver when an object needs a shared pointer to itself. But why would an object require that?
Use Cases for BOOST::ENABLE_SHARED_FROM_THIS
- Callbacks: When an object registers a callback and wants to ensure it’s not deleted before the callback is invoked;
- Shared Ownership: When part of an object’s functionality is offloaded to another object, but they need shared ownership of resources.
Implementing BOOST::ENABLE_SHARED_FROM_THIS
Remember, to use this feature, the class should inherit from boost::enable_shared_from_this<ClassType>.
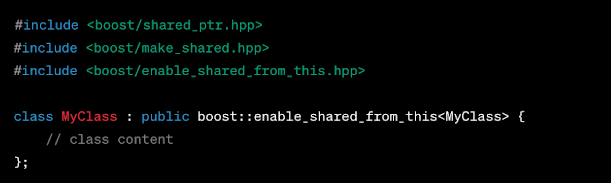
The Magic Behind The Scenes
When you dive into the underpinnings, you realize that BOOST::ENABLE_SHARED_FROM_THIS is not magical, but sheer brilliance.
How does it work?
Every time a shared pointer takes ownership of an object, it checks if the object inherits from boost::enable_shared_from_this. If yes, it updates an internal weak pointer with the shared pointer’s information.
Pitfalls & Precautions
While powerful, there are areas where one should tread cautiously:
- Never Use new Directly: Always use boost::make_shared to create shared pointers. This ensures the enable_shared_from_this functionality is correctly initialized;
- Beware of this Pointer: Directly using the this pointer can lead to unexpected behavior. Always prefer the shared_from_this function.
Comparing with Standard Library
With C++11 and beyond, the standard library also introduced shared pointers and the std::enable_shared_from_this feature.
Feature | Boost Library | Standard Library Library |
---|---|---|
Header File | boost/shared_ptr.hpp | memory |
enable_shared_from_this Class | boost::enable_shared_from_this | std::enable_shared_from_this |
Performance | Slightly slower due to legacy support | Faster in most cases due to modern optimizations |
Real-world Applications
You might wonder, “Where’s this used in the real world?” From game development to high-frequency trading systems, the applications are manifold.
Game Development:
Objects in a game, like characters or weapons, might need shared ownership to manage resources like textures or AI behavior modules.
Trading Systems:
In financial software, multiple subsystems might need shared access to market data, ensuring that data remains consistent and updated across all subsystems.
Circular Linked List in C
A detour from the Boost library, but an important concept nonetheless, is the Circular Linked List. Predominantly used in computer science and software engineering, it’s a type of linked list where the last node points back to the first node instead of having a null reference.
Defining the Structure
In the C programming language, a circular linked list node can be defined as:

Characteristics & Uses
- Endless Traversal: Unlike simple linked lists, you can traverse a circular linked list indefinitely;
- Applications: Useful in scenarios like a round-robin scheduling algorithm, where tasks are cycled through repeatedly.
Inserting a Node
Inserting a node requires checking if the list is empty. If it is, the new node becomes the head and points to itself. Otherwise, you traverse to the end and adjust pointers.
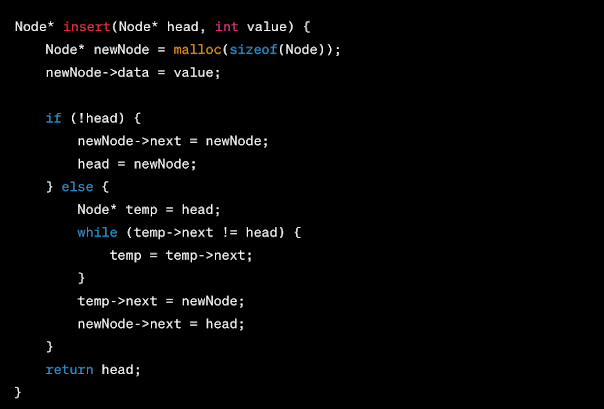
Advantages over Simple Linked List
- Continuous Access: You don’t need to backtrack to the head node after reaching the end, allowing for continuous access to elements;
- Space Utilization: Useful in environments like embedded systems, where space optimization is crucial.
Challenges
- Complex Deletion: Deleting a node, especially the last one, requires careful pointer adjustments to ensure the circular property remains intact;
- Infinite Loop Risk: If not handled properly, operations on the list can lead to infinite loops.
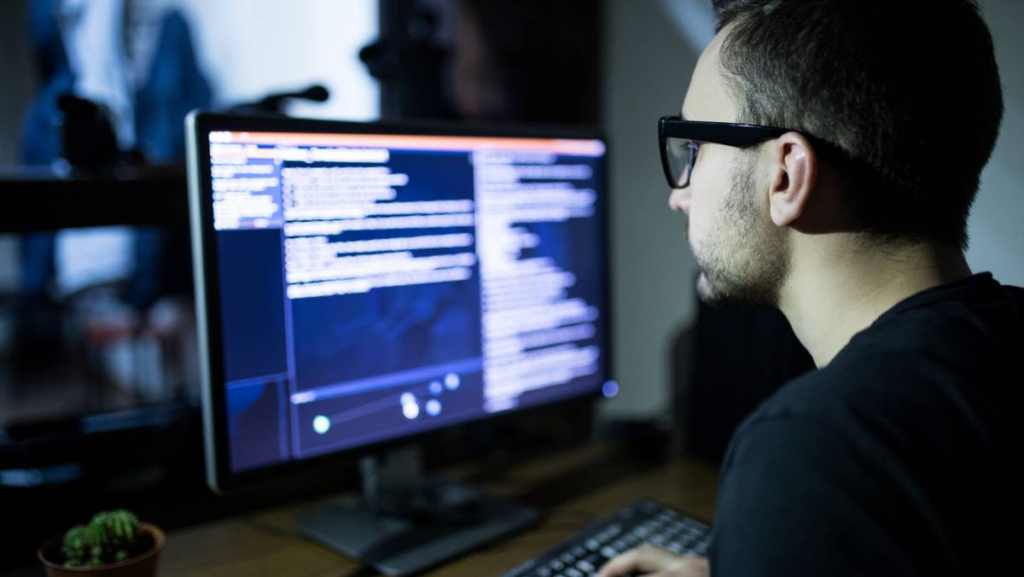
Conclusion
The BOOST::ENABLE_SHARED_FROM_THIS functionality, while just a cog in the vast Boost library, carries immense significance. It elegantly solves problems related to shared ownership, ensuring smoother, more efficient C++ code. As developers, understanding and implementing such features can make our code robust, maintainable, and efficient.
Frequently Asked Questions
It allows objects to safely create shared pointers to themselves.
Yes, the standard library has a similar feature named std::enable_shared_from_this.
No, it’s designed explicitly for shared pointers.
It can lead to undefined behavior, especially if there’s no existing shared pointer to the object.